What is the MVVM pattern and how is it implemented in Xamarin applications?
Model Answer
A strong candidate might describe the Model-View-ViewModel (MVVM) pattern as a design pattern used to separate the graphical user interface (UI) of an application from the business logic or back-end logic. In Xamarin, the Model manages the data and business logic, View handles the display, and ViewModel acts as the intermediary that binds the UI to the Model. Data binding between the View and ViewModel allows changes in data to be immediately reflected in the UI and vice versa.
Example
For instance, a candidate may have implemented MVVM by creating a ViewModel class that handles the data and command logic for a page, binding properties and commands to the Xamarin.Forms UI controls using XAML binding.
What Hiring Managers Should Pay Attention To
- Understanding of MVVM pattern in Xamarin.
- Experience in data binding and command usage.
- Ability to explain the separation of components in MVVM.
Can you describe the differences between Xamarin.Forms and Xamarin.Native (Xamarin.iOS and Xamarin.Android)?
Model Answer
A proficient candidate should explain that Xamarin.Forms is a cross-platform UI toolkit that allows developers to create user interfaces that can be shared across iOS, Android, and Windows. It’s ideal for applications with similar UI on multiple platforms. In contrast, Xamarin.Native uses platform-specific APIs to build applications with the platform's native UI. This approach provides greater control over each platform's unique features and user experience.
Example
In a project where performance was crucial, I chose Xamarin.Android for the Android-specific UI to leverage complex animations that Xamarin.Forms couldn't handle efficiently.
What Hiring Managers Should Pay Attention To
- Understanding of cross-platform vs native-specific advantages.
- Ability to decide when to use Xamarin.Forms over Xamarin.Native based on project requirements.
- Awareness of platform-specific features.
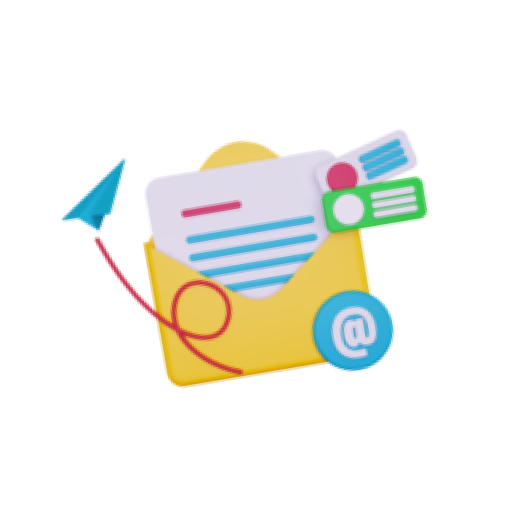
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Explain how you approach managing memory in Xamarin to prevent memory leaks.
Model Answer
A competent candidate should discuss detecting and resolving memory leaks by profiling the application, using weak references, and correctly unsubscribing event handlers. They could also mention utilizing Dispose patterns for unmanaged resources and monitoring memory consumption with tools like Xamarin Profiler.
Example
In a past project, I identified a memory leak caused by event handlers not being unsubscribed. I implemented weak event patterns and used Xamarin Profiler to ensure previous objects were properly disposed of, resulting in improved application performance.
What Hiring Managers Should Pay Attention To
- Awareness of common memory issues in mobile development.
- Experience with memory optimization techniques.
- Proficiency with profiling tools.
What strategies do you use to optimize app performance in Xamarin projects?
Model Answer
An apt response might include identifying performance bottlenecks through profiling, using async calls efficiently, and leveraging Xamarin.Forms visual states or compiled bindings. They could also suggest minimizing the use of conditional statements in rendering paths and utilizing preloading techniques for heavy resources.
Example
To optimize a Xamarin.Forms app, I reduced rendering latency by implementing compiled bindings and preloading images, coupled with cleaning up unused views to improve startup performance.
What Hiring Managers Should Pay Attention To
- Understanding of performance bottlenecks.
- Experience with both profiling and improving performance.
- Ability to apply Xamarin.Forms optimizations effectively.
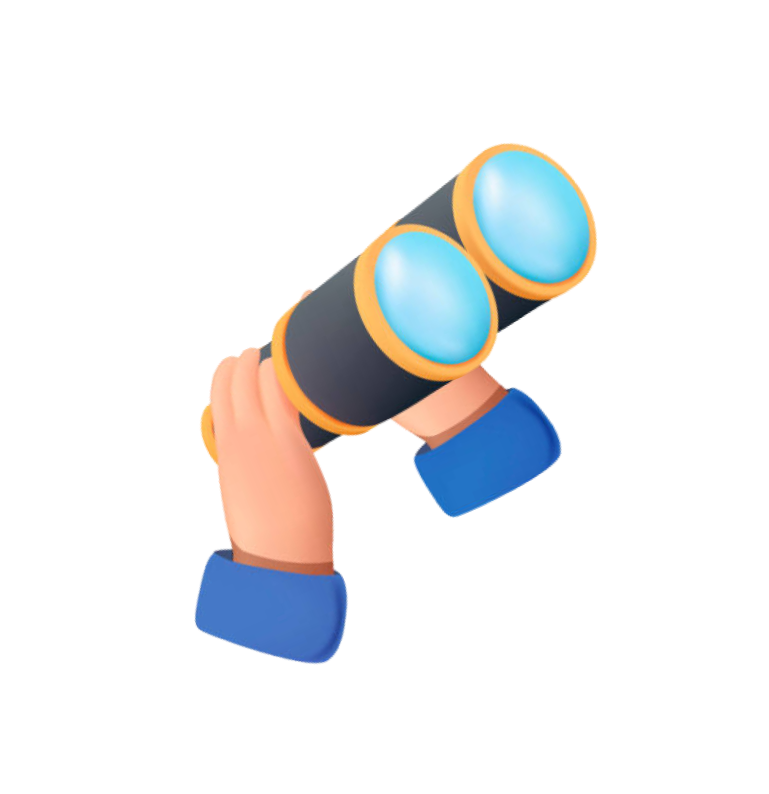
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
How do you implement CI/CD processes for Xamarin applications?
Model Answer
A candidate might describe setting up a CI/CD pipeline using tools like Azure DevOps or Jenkins, featuring automated build, testing, and deployment processes. The explanation should include branch management strategies and handling versioning to ensure a smooth deployment cycle.
Example
I set up a CI/CD pipeline where every commit triggered a build process that ran automated tests and created a deployable package, using Azure DevOps to streamline testing feedback and deployment.
What Hiring Managers Should Pay Attention To
- Knowledge of setting up CI/CD pipelines.
- Familiarity with automation tools in mobile development.
- Understanding of version control and automated testing importance.
Behavioral Question for Mid-Level Candidates
Describe a time when you had to address a significant bug in production. How did you handle it?
Model Answer
A solid candidate might recount recognizing the bug's impact, prioritizing its fix, communicating with stakeholders about the issue and expected resolution timeline, and implementing a hotfix while working on a more permanent solution.
Example
Once, a critical bug affecting user login was identified. I quickly set up a meeting to analyze the root cause and coordinated with my team to deploy a hotfix within hours, maintaining open communication with stakeholders throughout the process until a permanent update was released.
What Hiring Managers Should Pay Attention To
- Problem-solving skills.
- Responsiveness to urgent issues.
- Ability to communicate effectively with stakeholders.
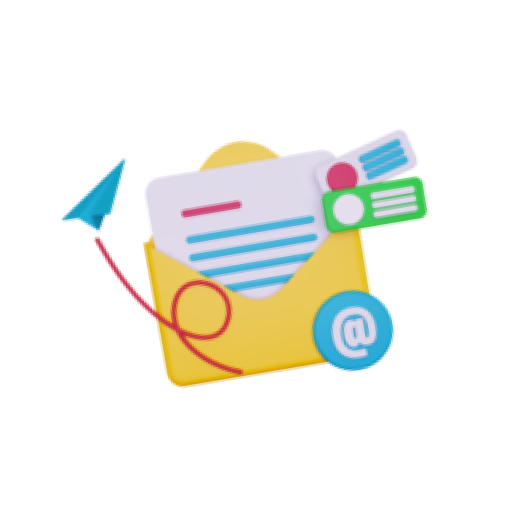
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Soft-Skills Questions for Mid-Level Candidates
How do you manage team collaboration in a remote work environment?
Model Answer
A strong candidate might describe the Model-View-ViewModel (MVVM) pattern as a design pattern used to separate the graphical user interface (UI) of an application from the business logic or back-end logic. In Xamarin, the Model manages the data and business logic, View handles the display, and ViewModel acts as the intermediary that binds the UI to the Model. Data binding between the View and ViewModel allows changes in data to be immediately reflected in the UI and vice versa.
Example
While working remotely, I scheduled daily stand-ups via Zoom and used Microsoft Teams to keep constant communication. I ensured all code reviews and feature descriptions were well-documented on Confluence for transparency.
What Hiring Managers Should Pay Attention To
- Use of communication and documentation tools.
- Ability to maintain team collaboration remotely.
- Setting clear goals and expectations.
How do you design an architecture for a complex Xamarin application?
Model Answer
An experienced candidate should elaborate on selecting suitable architectures like MVVM, Clean Architecture, or MVI based on the project needs. They should discuss structuring projects into self-contained modules, defining clear interfaces for components, and ensuring scalability and maintainability.
Example
For designing a financial application, I chose a Clean Architecture to separate concerns and make the core logic independent of UI layers, enabling easier testing and future scalability.
What Hiring Managers Should Pay Attention To
- Experience with architectural design patterns.
- Ability to explain the rationale behind choosing specific architectures.
- Understanding of scalability and maintainability principles.
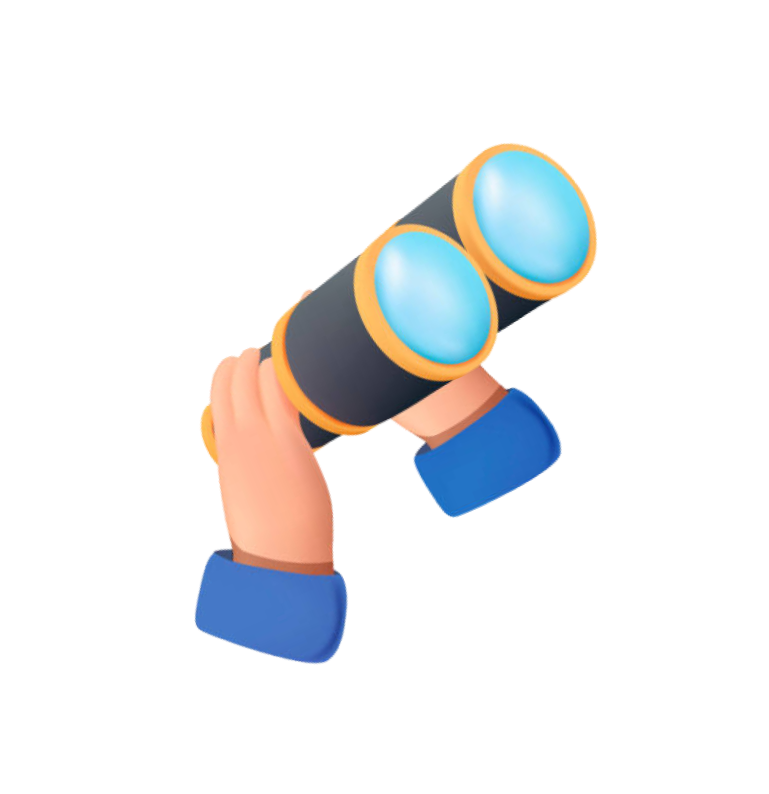
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Explain your experience with integrating third-party APIs in Xamarin apps.
Model Answer
The candidate should discuss evaluating APIs for reliability and performance, managing network calls with HttpClient or third-party libraries like Refit, handling authentication, and ensuring responsive UI with async data fetching. They should also mention adhering to security best practices when storing API keys.
Example
In developing a travel app, I integrated the Google Maps API using Refit for cleaner network abstraction, implemented OAuth 2.0 for secure API calls, and ensured all responses were processed asynchronously to maintain UI responsiveness.
What Hiring Managers Should Pay Attention To
- Experience with API integration and security.
- Familiarity with libraries and network call management.
- Ability to implement async data processing effectively.
How do you ensure code quality and maintainability in your projects?
Model Answer
An experienced candidate might discuss implementing code review processes, utilizing static code analysis tools, adhering to SOLID principles, and consistently writing unit and integration tests. They should emphasize fostering a culture of continuous improvement within the team.
Example
In a team-setting project, I established a code review protocol using tools like SonarQube for static analysis and enforced a Git branching strategy, which helped to catch defects early and maintain a high standard of code quality.
What Hiring Managers Should Pay Attention To
- Experience with code quality and review processes.
- Knowledge of static analysis and testing tools.
- Commitment to maintaining high coding standards.
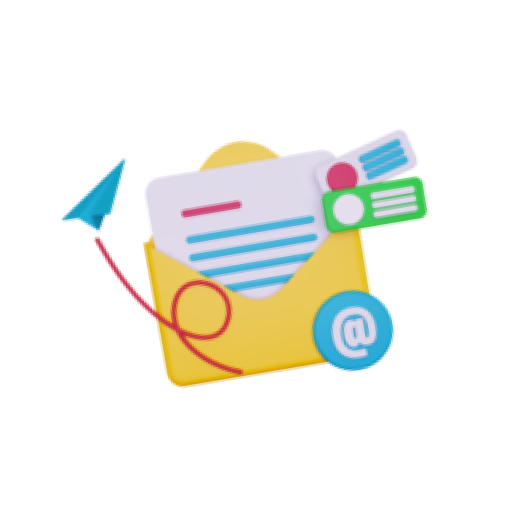
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Behavioral Question for Senior-Level Candidates
Share an example of a major project you led. What was the outcome?
Model Answer
A competent candidate should detail setting objectives, coordinating resources, planning and executing project strategies, and overcoming challenges. They should highlight the results achieved and lessons learned, reflecting on how these experiences influenced future project leadership approaches.
Example
I led the development of an organizational app which involved cross-functional teams. Through effective planning and execution, we achieved the project's objectives ahead of schedule, enhancing user engagement metrics by 30% within six months post-launch.
What Hiring Managers Should Pay Attention To
- Project management and leadership skills.
- Experience in delivering impactful outcomes.
- Ability to reflect and learn from past projects.
Soft-Skills Questions for Senior-Level Candidates
How do you mentor junior developers in your team?
Model Answer
The candidate should describe creating a supportive environment for learning, offering regular feedback, setting achievable goals, encouraging involvement in design discussions, and guiding them through code reviews. They might also highlight creating knowledge-sharing sessions or pair programming as part of their mentoring strategy.
Example
I organized weekly knowledge-sharing sessions and paired junior developers with more experienced ones on key projects to promote skill enhancement and team integration.
What Hiring Managers Should Pay Attention To
- Leadership and mentoring skills.
- Ability to foster a learning environment.
- Commitment to team development.
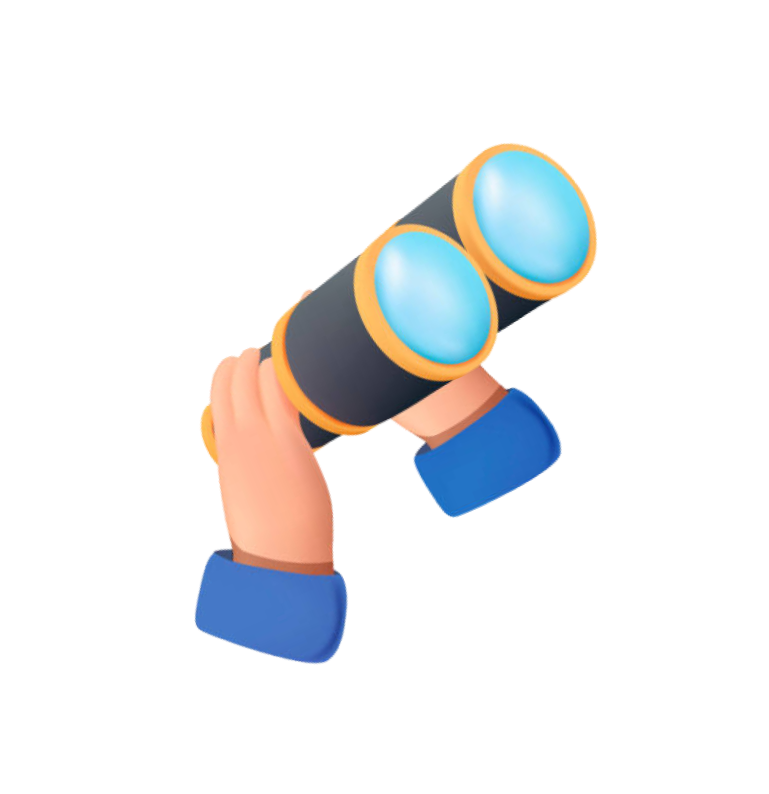
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process