What are the differences between a list and a tuple in Python?
Model Answer
A list in Python is a mutable data structure, meaning it can be changed after its creation, allowing appending, removing, or changing items. A tuple, on the other hand, is immutable, so its content cannot be altered once defined. Lists are defined with square brackets [], while tuples use parentheses (). Use a list when data is expected to change; use a tuple when it is constant.
Example
In a project, I used a tuple to store the dimensions of a rectangle since these were fixed values, whereas I used a list for storing items to be processed, as this list was updated frequently.
What Hiring Managers Should Pay Attention To
- Understanding of mutable vs. immutable data structures
- Correct usage scenarios for lists and tuples
- Ability to clearly articulate differences
Explain the concept of Python decorators and provide an example use case.
Model Answer
Decorators in Python are a way to modify or extend the behavior of functions or methods without changing their code. They wrap another function, often used to add logging, authorization, or timing functionality. The '@' symbol is used for applying a decorator to a function.
Example
For instance, I created a logging decorator to automatically log the execution time of several functions during a performance-sensitive project.
What Hiring Managers Should Pay Attention To
- Clear explanation of decorators
- Understanding of practical use cases
- Ability to provide a relevant example
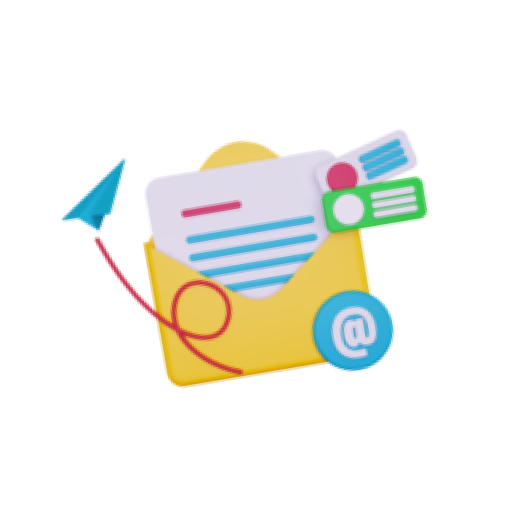
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Explain the concept of list comprehensions and how they can be used efficiently in Python.
Model Answer
List comprehensions provide a concise way to create lists in a single line, typically offering better readability and performance. They consist of brackets containing an expression followed by a for clause, and optionally more for or if clauses.
Example
I used list comprehensions to quickly generate a list of squared numbers from another list, improving code simplicity and performance.
What Hiring Managers Should Pay Attention To
- Understanding of the syntax
- Ability to articulate benefits
- Examples of improved code efficiency
How do you optimize Python code for performance? Give some strategies you have used.
Model Answer
To optimize Python code, one might profile the code to find bottlenecks, use more efficient data structures and algorithms, apply caching techniques, or leverage the concurrency and parallelism capability through modules like asyncio or multiprocessing.
Example
I optimized a data processing script by switching from list to set operations, significantly reducing time complexity when handling large datasets.
What Hiring Managers Should Pay Attention To
- Knowledge of profiling tools
- Understanding of efficient data structures
- Clear examples of past optimizations
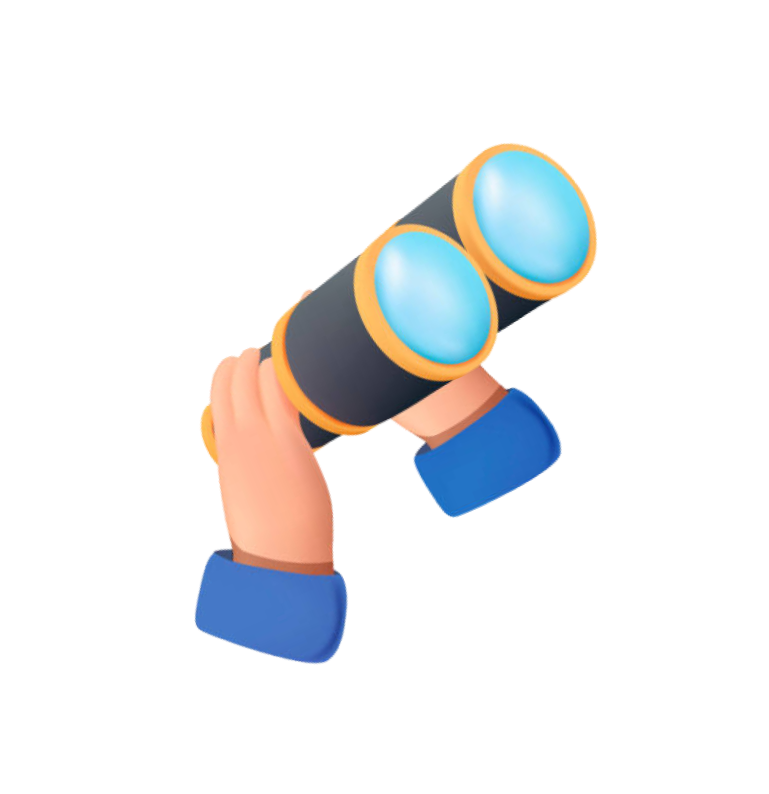
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
What is a Python generator, and how is it different from a list comprehension?
Model Answer
A generator in Python is a function that yields results one at a time and only as needed, which is more memory efficient than generating a whole list at once. Unlike list comprehensions, generators use the yield statement and can handle large data sequences without significant memory use.
Example
I implemented a generator to process a large log file, yielding lines one at a time to minimize memory usage.
What Hiring Managers Should Pay Attention To
- Understanding of yield vs. return
- Memory management efficiency
- Real-world use cases
Behavioral Question for Mid-Level Candidates
Can you discuss a time when you had to advocate for a technical change in a project?
Model Answer
A good response would describe identifying a need for change, compiling supporting data or examples, presenting the case to stakeholders, and addressing concerns or resistance effectively.
Example
In a recent role, I advocated for adopting a new testing framework by demonstrating its benefits in terms of coverage and efficiency, ultimately gaining buy-in from the team.
What Hiring Managers Should Pay Attention To
- Influence without authority
- Data-driven decision-making
- Ability to handle resistance
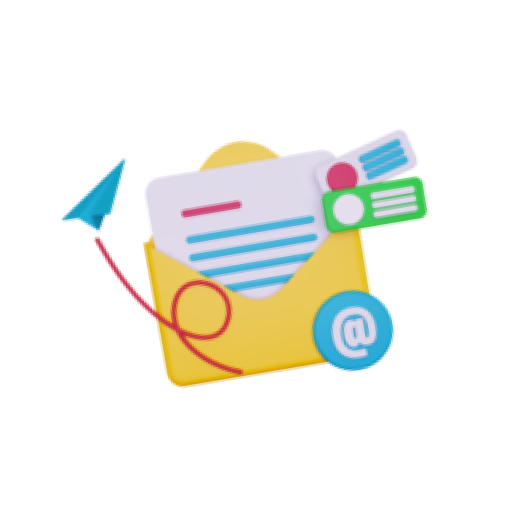
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Soft-Skills Questions for Mid-Level Candidates
What strategies do you employ to stay current with the latest Python trends and technologies?
Model Answer
A list in Python is a mutable data structure, meaning it can be changed after its creation, allowing appending, removing, or changing items. A tuple, on the other hand, is immutable, so its content cannot be altered once defined. Lists are defined with square brackets [], while tuples use parentheses (). Use a list when data is expected to change; use a tuple when it is constant.
Example
I regularly follow the Python Enhancement Proposals and contribute to open-source projects on GitHub to stay updated.
What Hiring Managers Should Pay Attention To
- Ongoing learning commitment
- Participation in community events
- Proactivity in skill development
Describe how you approached building a scalable Python application.
Model Answer
An effective approach involves utilizing modular architecture, leveraging asynchronous methods for I/O-bound tasks, optimizing code using profiling tools, and ensuring thorough testing. Choosing cloud services for scalability and employing containerization tools can also be part of the strategy.
Example
In a recent project, I refactored the application to use microservices architecture and implemented Docker for easier scaling and deployment.
What Hiring Managers Should Pay Attention To
- Experience with scalable architectures
- Use of modern tools (e.g., Docker)
- Ability to articulate technical decisions
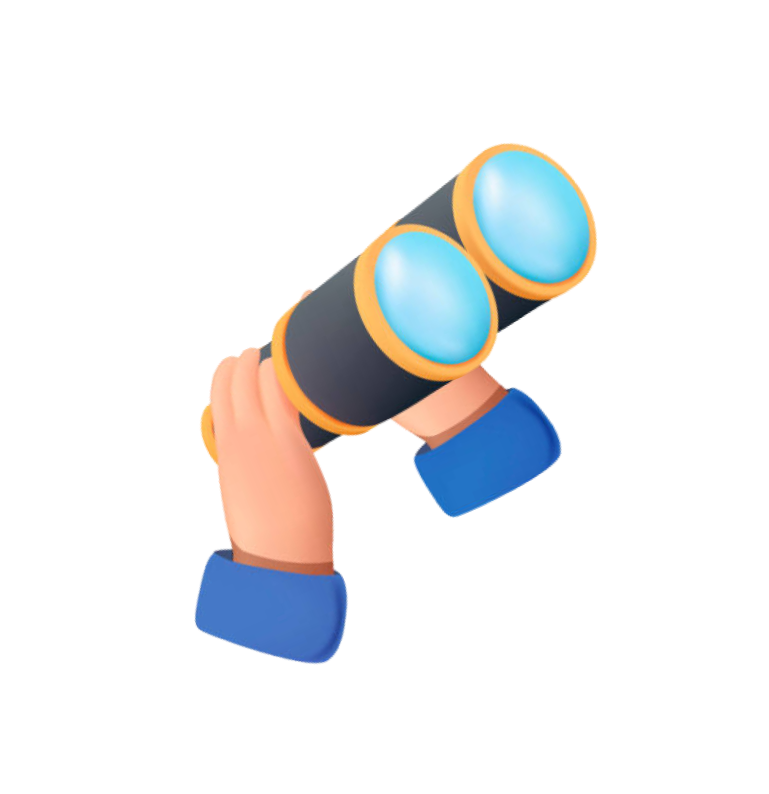
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
What is your experience with integrating Python applications with other technologies or systems?
Model Answer
A strong candidate will have experience with APIs, databases, and other programming languages, and should describe the methods and tools they used for successful integrations, such as RESTful services or ORM libraries.
Example
I integrated a Python application with a Java-based system using REST APIs, facilitating seamless data exchange between the systems.
What Hiring Managers Should Pay Attention To
- Variety of integration experience
- Familiarity with relevant technologies
- Successful integration examples
How do you ensure your team adheres to best programming practices in Python?
Model Answer
Ensuring adherence to best practices involves setting up a code review process, using linters and adherent tools for code standardization, and organizing regular technical meetings or workshops.
Example
I implemented a code review system where team members submit pull requests for peer review, ensuring adherence to PEP 8 guidelines before merging changes.
What Hiring Managers Should Pay Attention To
- Leadership in technical standardization
- Implementation of review processes
- Use of automation tools for quality control
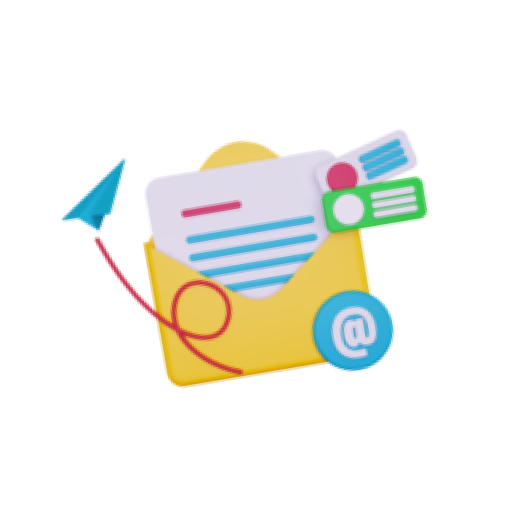
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Behavioral Question for Senior-Level Candidates
Discuss a challenging technical problem you faced and how you resolved it.
Model Answer
A strong candidate will explain the context, the steps taken to understand the problem, the collaborative efforts if involved, and the solution they implemented. They should highlight any innovative or persistent approaches they utilized.
Example
In a high-volume transaction system, I encountered a performance bottleneck which I resolved by profiling the code to identify the issue and then implemented a caching mechanism, reducing response time significantly.
What Hiring Managers Should Pay Attention To
- Problem-solving skills
- Innovative thinking
- Collaboration and resilience
Soft-Skills Questions for Senior-Level Candidates
How do you inspire and lead a team of developers towards a common project goal?
Model Answer
Articulate a vision for the project, communicate clearly, build rapport, recognize individual contributions, and foster an environment of collaboration and continuous feedback. Regular check-ins and setting progressive milestones can keep the team motivated and focused.
Example
I held regular stand-ups and retrospectives to ensure the team was aligned on objectives and addresses obstacles promptly, resulting in high team morale and project success.
What Hiring Managers Should Pay Attention To
- Visionary leadership
- Effective communication skills
- Fostering a collaborative environment
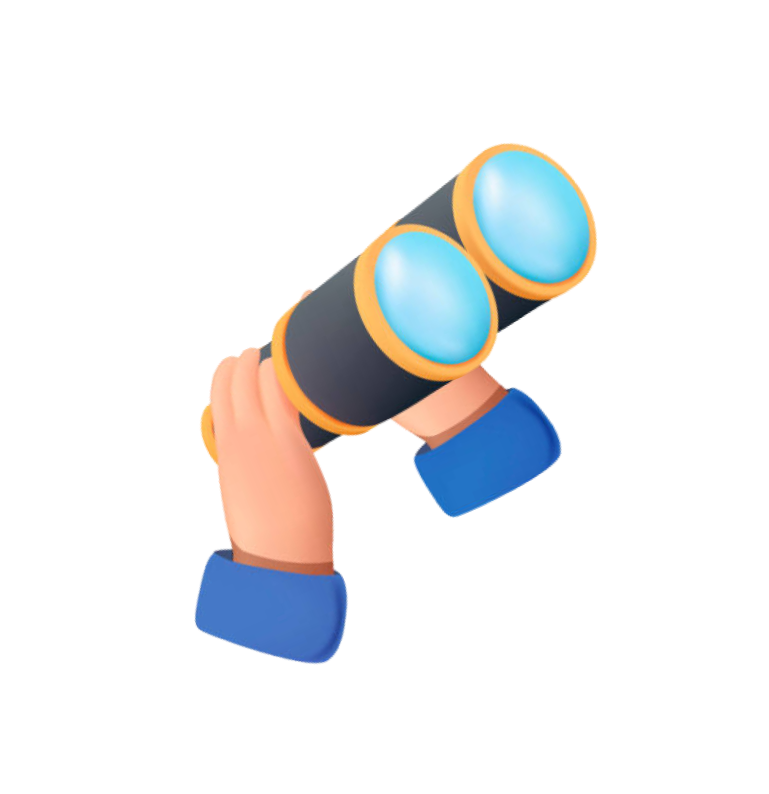
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process