What is the event loop in Node.js and how does it work?
Model Answer
A strong candidate should explain that the event loop is a crucial part of Node.js that allows it to perform non-blocking I/O operations. They should mention how Node.js handles asynchronous operations by placing tasks on an event queue and then processing them in a loop. The event loop continually checks for completed tasks and executes their callbacks, allowing for efficient single-threaded, non-blocking task management.
Example
For example, when a Node.js server receives HTTP requests, it uses the event loop to handle multiple incoming requests without creating a new thread for each request.
What Hiring Managers Should Pay Attention To
- Understanding of fundamental Node.js concepts
- Ability to summarize complex processes succinctly
- Grasp of asynchronous operations and their benefits
Explain how you can handle errors in Node.js applications.
Model Answer
A good response would cover techniques such as try-catch blocks, using error event listeners for asynchronous calls, and strategies for promise rejections. They might also discuss logging errors for later analysis and notification systems to alert developers of critical failures.
Example
Once, I used try-catch to wrap a function that made several database calls. If any call failed, it would log the error details and send an alert to the responsible developer.
What Hiring Managers Should Pay Attention To
- Knowledge of error-handling mechanisms
- Understanding the importance of logging and alerts
- Appreciation of best practices in maintaining application stability
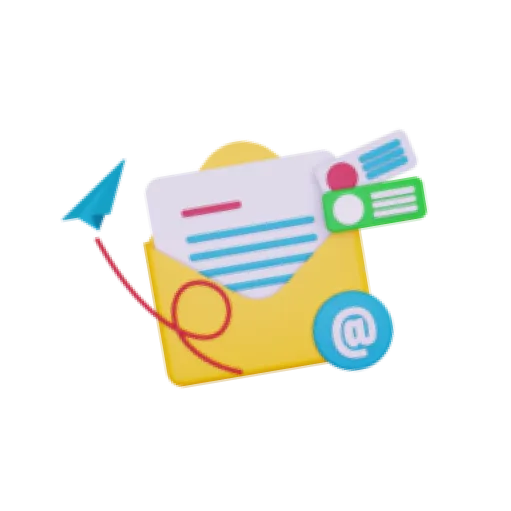
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
How do you optimize the performance of a Node.js application?
Model Answer
A well-rounded answer might include strategies such as profiling the application to identify bottlenecks, employing clustering for load balancing, using asynchronous code effectively, and optimizing database queries. They may also mention tools like PM2 or NGiNX for better load management.
Example
In a past project, I used PM2 to cluster application instances, reducing response times by efficiently handling incoming requests. I also optimized database queries by adding indexes to frequently queried fields.
What Hiring Managers Should Pay Attention To
- Understanding of performance bottlenecks
- Familiarity with tools and techniques for optimization
- Ability to articulate specific examples of performance improvement
- Strategic thinking in resource allocation
Can you explain how middleware works in Express.js and give an example of its use?
Model Answer
The candidate should articulate that middleware functions in Express.js are executed sequentially and can perform actions on request and response objects. They should provide examples such as logging, authentication, and error handling, illustrating how middleware adds modularity to applications.
Example
I implemented an authentication middleware that checks for a valid token before allowing access to protected routes and logs unauthorized attempts for audit trails.
What Hiring Managers Should Pay Attention To
- Understanding of Express.js middleware
- Ability to integrate utility functions efficiently
- Problem-solving skills with regard to application structure
- Use of middleware for clean, maintainable code

Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Discuss the differences between process.nextTick() and setImmediate() in Node.js.
Model Answer
They should explain that process.nextTick() defers the execution of a callback until after the current operation completes, before any I/O events are fired. In contrast, setImmediate() schedules the callback to execute on the next iteration of the event loop, after any I/O events.
Example
I used process.nextTick() in a function to quickly execute a dependent callback without waiting for the next event loop, thus improving responsiveness in a high-performance application.
What Hiring Managers Should Pay Attention To
- Understanding of the event loop and execution order
- Clear explanation of timing and execution differences
- Ability to choose the right method based on context and performance needs
Behavioral Question for Mid-Level Candidates
Tell me about a time you had to handle a critical production issue. What was the outcome?
Model Answer
The candidate should describe the incident troubleshooting process, including identifying the issue, the steps taken to resolve it, and how they communicated the situation to stakeholders. They should emphasize their ability to remain calm under pressure and quickly implement a solution to minimize downtime.
Example
While handling a production issue where the API services went down, I quickly identified a memory leak from an infinite loop, fixed the code, and pushed the update within an hour, keeping stakeholders updated throughout.
What Hiring Managers Should Pay Attention To
- Problem-solving under pressure
- Technical troubleshooting skills
- Clear, timely communication
- Effectiveness in crisis management
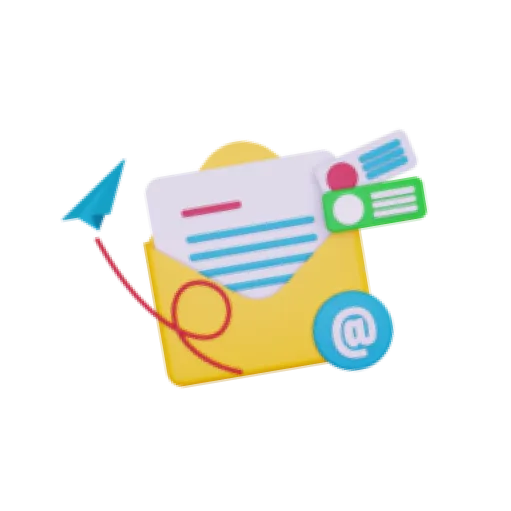
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Soft-Skills Questions for Mid-Level Candidates
Why do you think collaboration is important in software development, and how do you foster it?
Model Answer
A strong candidate should explain that the event loop is a crucial part of Node.js that allows it to perform non-blocking I/O operations. They should mention how Node.js handles asynchronous operations by placing tasks on an event queue and then processing them in a loop. The event loop continually checks for completed tasks and executes their callbacks, allowing for efficient single-threaded, non-blocking task management.
Example
In a team project, I promoted using Slack for on-the-spot communication and GitHub for collaborative coding, which ensured transparency and collaborative problem-solving.
What Hiring Managers Should Pay Attention To
- Value placed on teamwork and collaboration
- Experience with communication and collaboration tools
- Understanding of the benefits of collaborative environments
- Ability to lead or participate in team efforts
How do you approach designing the architecture for a large-scale Node.js application?
Model Answer
A suitable candidate should discuss considering factors like scalability, maintainability, and performance. They should describe their approach to modular design, microservices, RESTful or GraphQL API structures, database schema design, and the role of serverless functions if applicable. Security considerations and customer experience should also be included in the response.
Example
For a high-traffic e-commerce site, I used a microservices architecture with separate services for user management, order processing, and inventory handling, ensuring each could scale independently, reducing bottlenecks.
What Hiring Managers Should Pay Attention To
- Understanding of architectural principles
- Ability to balance trade-offs in design decisions
- Strategic planning and long-term consideration
- Emphasis on scalability and security

Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Can you explain a situation where you had to introduce a new technology or process to improve your Node.js development workflow?
Model Answer
The answer should include why the decision was made, how they evaluated the available options, and the impact of the change. They should discuss trials or tests conducted, stakeholder buy-in, and how they managed the shift, including training provided and retrospective evaluation of results.
Example
I introduced Docker to streamline our development and production environments, facilitating consistent deployments and easier scalability, improving overall project flow and collaboration quality.
What Hiring Managers Should Pay Attention To
- Open-mindedness to adopt new technologies
- Ability to evaluate and implement new processes
- Focus on continuous improvement
- Impact on team workflow and project outcomes
What strategies would you use to mentor junior Node.js developers on your team?
Model Answer
They should talk about providing structured learning paths, fostering an environment of open communication, and using pair programming to facilitate skill transfer. They might mention regular code reviews with constructive feedback, and setting up regular knowledge-sharing sessions.
Example
In my team, I allocate time for junior developers to engage in pair programming on complex tasks and conduct bi-weekly knowledge-sharing sessions to discuss new findings and improve overall team skills.
What Hiring Managers Should Pay Attention To
- Mentorship and teaching capability
- Patience and empathy
- Ability to provide clear, constructive feedback
- Focus on skill development and team growth
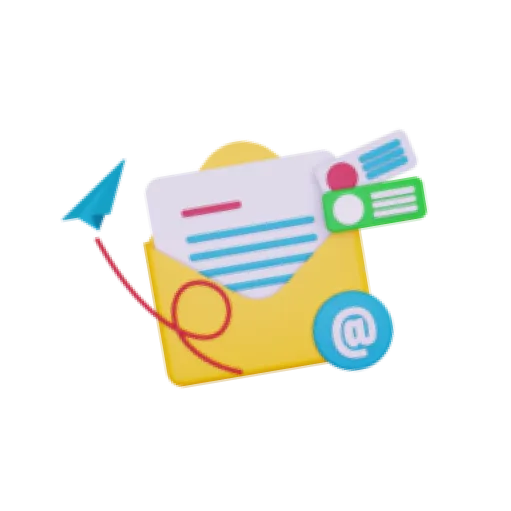
Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process
Behavioral Question for Senior-Level Candidates
Share an example of a time when you had to lead a team through a challenging project.
Model Answer
The answer should focus on how they understood the project's challenges, devised a plan, motivated the team, and ensured resources were adequately utilized. Mentioning ongoing communication, risk management, and how they handled setbacks positively would round out the example.
Example
Leading a migration to a new database system under a tight timeline, I established clear objectives, organized tasks efficiently, maintained morale through regular updates, and managed unforeseen issues by reallocating resources to critical paths.
What Hiring Managers Should Pay Attention To
- Leadership and motivation skills
- Ability to plan and execute effectively
- Problem-solving and adaptability
- Communication and resource management
Soft-Skills Questions for Senior-Level Candidates
How do you handle feedback from peers or subordinates, especially if it's not positive?
Model Answer
A model answer involves actively listening to the feedback, assessing its validity, and responding professionally. They should outline reviewing their actions if necessary, making adjustments, and thanking the individual for their perspective. It reflects a mature attitude towards personal and professional growth.
Example
When a peer pointed out that my decision-making could be more inclusive, I arranged a team meeting to open up discussions, sought broader inputs for choices, and thanked them for highlighting this area of improvement.
What Hiring Managers Should Pay Attention To
- Openness to feedback and self-improvement
- Emotional intelligence
- Professionalism in accepting criticism
- Proactive use of feedback for growth

Professionally crafted, role-specific questions
Covers technical, behavioral, and situational assessments
Helps streamline and improve your hiring process